◾️ Django forms
- create forms.py
from django import forms
from django_countries.fields import CountryField
from . import models
class SearchForm(forms.Form):
# https://docs.djangoproject.com/en/3.2/ref/forms/api/
# https://docs.djangoproject.com/en/3.2/ref/forms/fields/
# https://pypi.org/project/django-countries/
city = forms.CharField(initial="Anywhere")
country = CountryField(default="JP").formfield()
price = forms.IntegerField(required=False)
room_type = forms.ModelChoiceField(
empty_label="Any kind", queryset=models.RoomType.objects.all(), required=False
)
price = forms.IntegerField(required=False)
room_type = forms.ModelChoiceField(queryset=models.RoomType.objects.all())
guests = forms.IntegerField(required=False)
bedrooms = forms.IntegerField(required=False)
beds = forms.IntegerField(required=False)
baths = forms.IntegerField(required=False)
instant_book = forms.BooleanField(required=False)
superhost = forms.BooleanField(required=False)
amenities = forms.ModelMultipleChoiceField(queryset=models.Amenity.objects.all())
facilities = forms.ModelMultipleChoiceField(queryset=models.Facility.objects.all())
house_rules = forms.ModelMultipleChoiceField(
queryset=models.HouseRule.objects.all()
)
- views
以前情報維持:forms.SearchForm(request.GET)
init: unbound form : forms.SearchForm() without validation
after: bounded form : forms.SearchForm(request.GET)
def search(request):
print(request)
form = forms.SearchForm()
return render(request, "rooms/search.html", {"form": form})
- template
{% extends "base.html" %}
{% block page_title %}
Room Search | airbnb
{% endblock page_title %}
{% block search-bar %}
{% endblock search-bar %}
{% block content %}
<h2>Search</h2>
<h4>Searching by {{city}}</h4>
<form action="{% url "rooms:search" %}" method="get">
{{form.as_p}}
<button>Search</button>
</form>
<h3>Search Results</h3>
{% for r in rooms %}
<ul>
<li>
<h5>{{r.name}}</h5>
</li>
</ul>
{% endfor %}
{% endblock %}
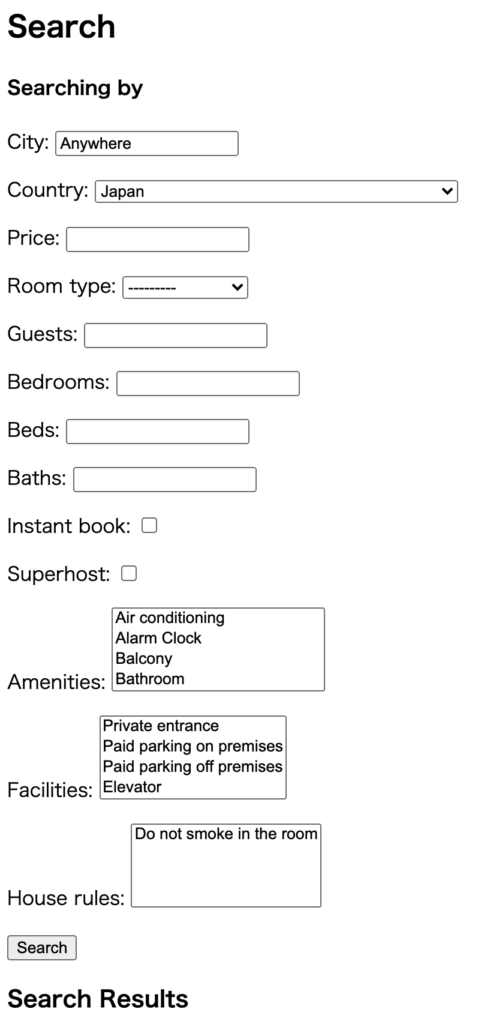
◾️ show checkbox for many to many field(amenities/facilities/house rules)
- use widget option(widget=forms.CheckboxSelectMultiple)
amenities = forms.ModelMultipleChoiceField(
queryset=models.Amenity.objects.all(), widget=forms.CheckboxSelectMultiple
)
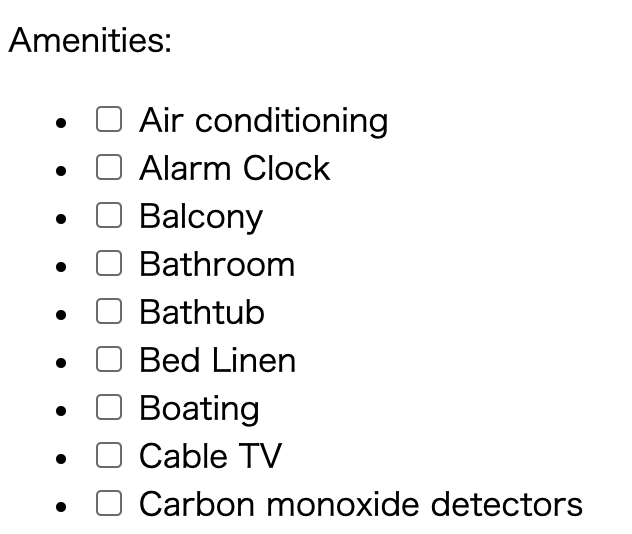