◾️ propTypes – check type(validator)
import PropTypes from "prop-types";
function Gallery({ id, name, image, rating }) {
return (
<div>
<h1>The Gallery Name is {name}</h1>
<h2>The Gallery Id is {id}</h2>
<h3>Rating is {rating}/5.0</h3>
<img src={image} alt={name} />
</div>
);
}
Gallery.propTypes = {
id: PropTypes.number.isRequired,
name: PropTypes.string.isRequired,
image: PropTypes.string.isRequired,
rating: PropTypes.number.isRequired,
};
◾️ axios – fetch data
getMovies = async () => {
const movies = await axios.get("https://yts-proxy.now.sh/list_movies.json");
};
◾️ react-youtube
import React from "react";
import Youtube from "react-youtube";
const VideoPlay = ({ id }) => {
console.log("VideoPlay:", id);
return (
<div>
<Youtube videoId={id} />
</div>
);
};
export default VideoPlay;
◾️ react-linkify :
React component to parse links (urls, emails, etc.) in text into clickable links
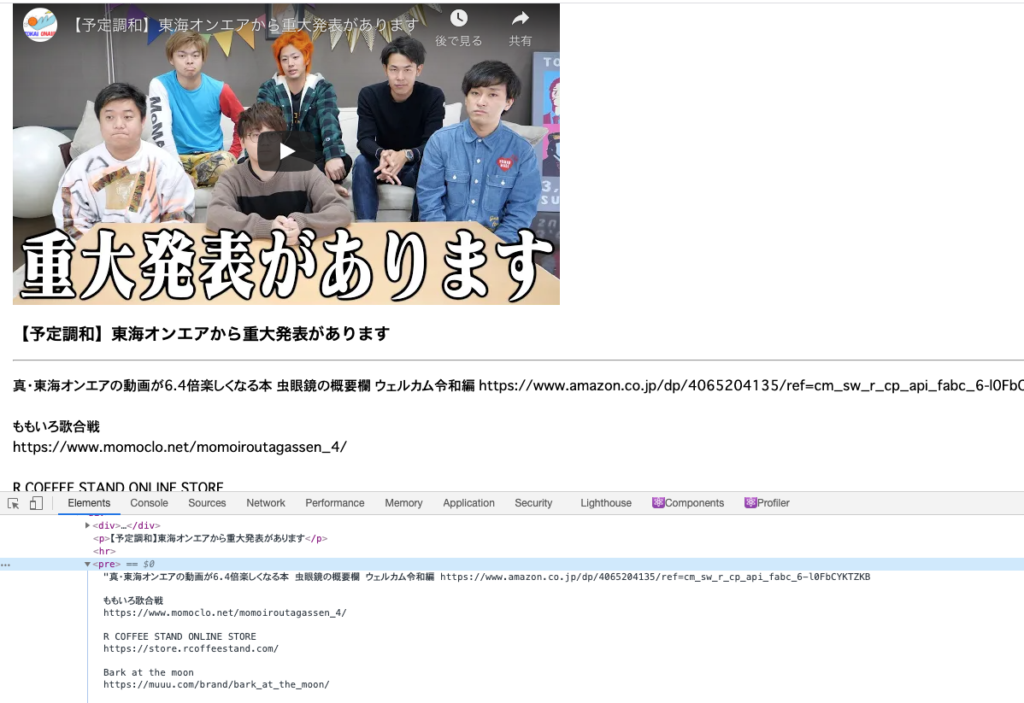
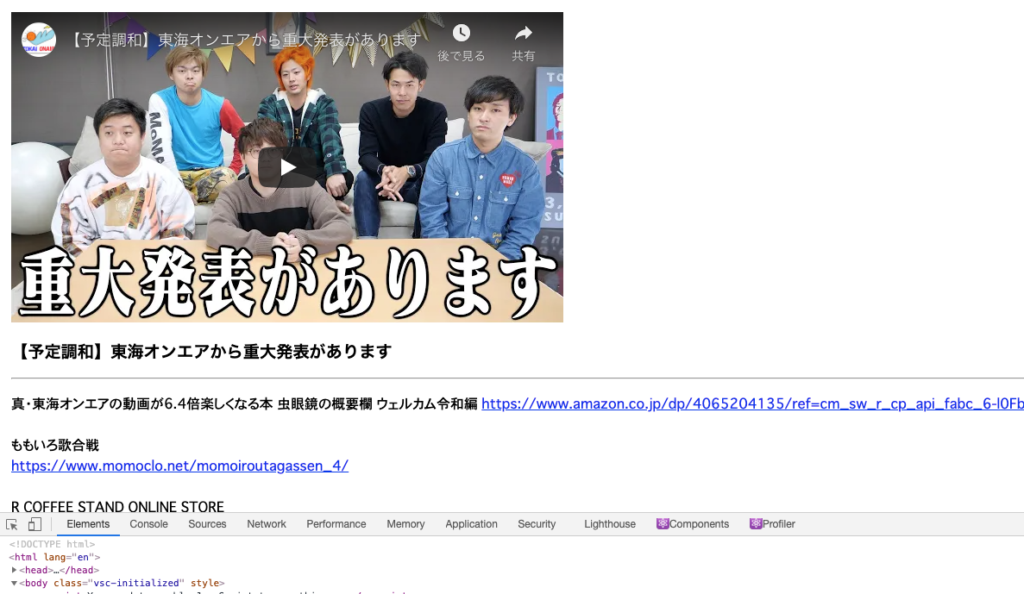